How to pass data from controller to blade view in laravel. This tutorial will cover on every methods which help us to pass the data form controller to our view blade file.
This tutorial is going to work with all version of laravel 5, laravel 6, laravel 7, laravel 8 and laravel 9. You can test the tutorial on the version let us know if there is any problem in the comment section.
There is a tutorial on Laravel 9 Get env Variable in Blade File Example you may also be interest on reading this article also.
We are going to deal with three ways to pass data from controller to blade view. The examples are as follow:
- Using Compact()
- Using Array
- Using With()
So let get started with each example one by one.
Also Read: Add Toast Notification in Laravel 9
Example 1: Using Compact()
The compact() method makes it a lot easier to parse data to the view in a readable format.
There are many methods to pass data, especially data queried from the database.
This shot explains how to use the compact() method to elegantly parse data to your views.
So let see the DemoController.php
app/Http/Controllers/DemoController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class DemoController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index(Request $request)
{
$title = "Welcome to LaravelTuts.com";
$subTitle = "Thank you";
return view('demo', compact('title', 'subTitle'));
}
}
The above example pass the title and subTitle to the blade view file using compact().
resources/views/demo.blade.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Pass Data from Controller to Blade View Laravel</title>
</head>
<body>
<h1>{{ $title }}</h1>
<h2>{{ $subTitle }}</h2>
</body>
</html>
That will display title and subTitle in view.
Also Read: Laravel 9 Shopping Cart Tutorial and Example
Example 2: Using Array
This example explain passing data to view using Array.
Let see the example for pass data from controller to blade view using Array.
app/Http/Controllers/DemoController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class DemoController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index(Request $request)
{
$title = "Welcome to LaravelTuts.com";
$subTitle = "Thank you";
return view('demo', [
'title' => $title,
'subTitle' => $subTitle,
]);
}
}
The above example pass the title and subTitle to the blade view file using Array.
resources/views/demo.blade.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Pass Data from Controller to Blade View Laravel</title>
</head>
<body>
<h1>{{ $title }}</h1>
<h2>{{ $subTitle }}</h2>
</body>
</html>
That will display title and subTitle in view.
Also Read: Laravel Add Watermark on Images
Example 3: Using With()
At last, we are passing data using with().
Let see the example on how to pass data from controller to blade view using with().
app/Http/Controllers/DemoController.php
<?php
namespace App\Http\Controllers;
use Illuminate\Http\Request;
class DemoController extends Controller
{
/**
* Write code on Method
*
* @return response()
*/
public function index(Request $request)
{
$title = "Welcome to LaravelTuts.com";
$subTitle = "Thank you";
return view('demo')
->with('title', $title)
->with('subTitle', $subTitle);
}
}
The above example pass the title and subTitle to the blade view file with().
resources/views/demo.blade.php
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Pass Data from Controller to Blade View Laravel</title>
</head>
<body>
<h1>{{ $title }}</h1>
<h2>{{ $subTitle }}</h2>
</body>
</html>
That will display title and subTitle in view.
Also Read: How to Check Date is Today’s Date or not in Laravel Carbon?
Let see the output for all above examples.
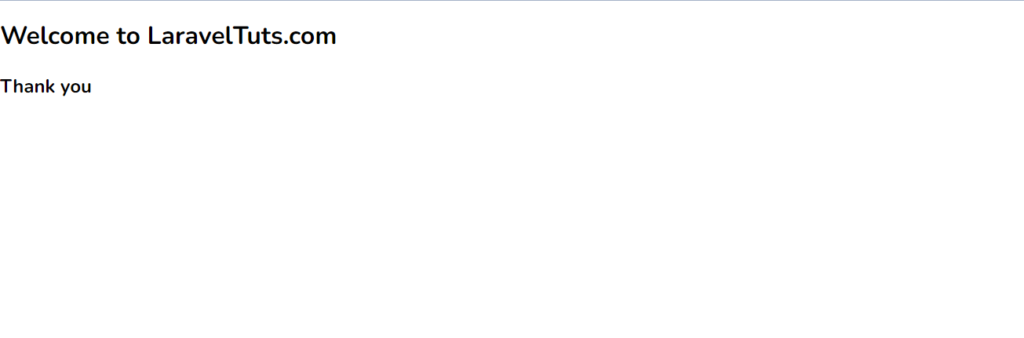
Conclusion
Today, We had learn Pass data from Controller to Blade View in Laravel. Hope this tutorial helped you with learning Laravel 9. If you have any question you can ask us at comment section below. If you like the tutorial please subscribe our YouTube Channel and follow us on social network Facebook and Instagram.
Also Read: Working with TMDb API in Laravel 9